React 19: Features, Updates, and How to Upgrade Your React Apps
Discover React 19's latest features, including Actions, Server Components, new hooks, and the React Compiler. Learn how these updates improve performance, enhance SEO, and simplify React development.
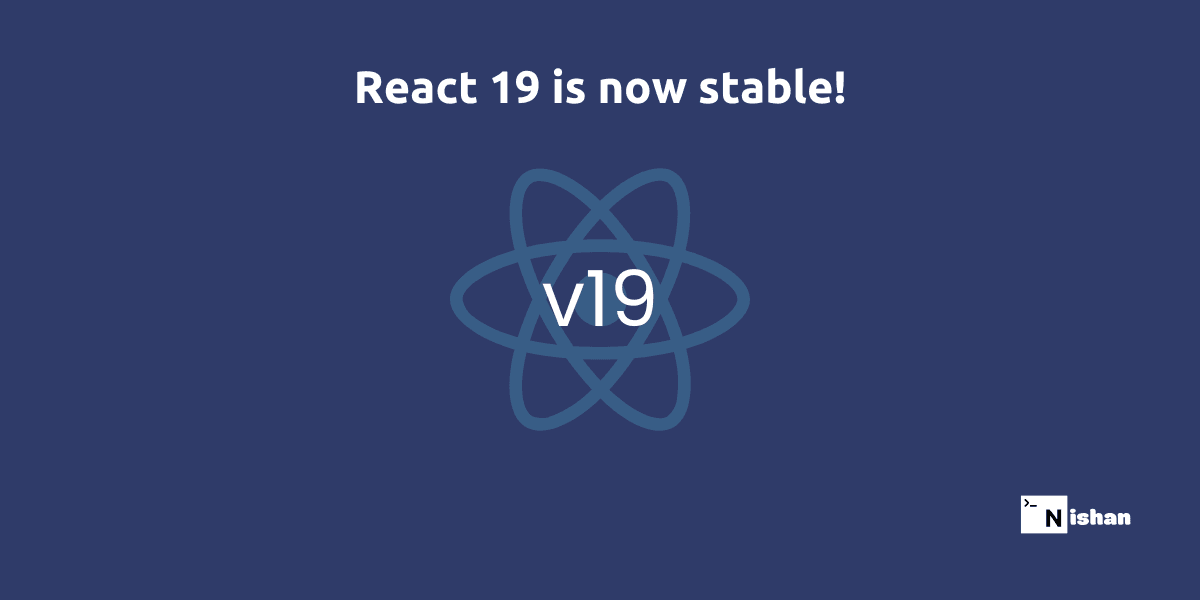
The React team has launched React 19, bringing powerful features to enhance performance, simplify development, and improve user experience. This release is packed with tools that streamline building modern web apps while keeping React as intuitive as ever.
If you’re a developer working with ReactJS, React Server Components, or looking to improve your React app performance, this guide will walk you through everything React 19 offers.
🚀 What’s New in React 19?
1. Introducing Actions: Simplifying State and Data Mutations
One of the standout features in React 19 is Actions, designed to make asynchronous operations like form submissions and data mutations easier.
Why Actions are game-changing:
- No need for manual state management during async operations.
- Automatically handles loading states, errors, and optimistic updates (i.e., showing the update before server confirmation).
Here’s an example:
"use client";
import { useFormStatus } from "react";
function SubmitButton() {
const { pending } = useFormStatus();
return <button disabled={pending}>Submit</button>;
}
With Actions, React takes care of everything behind the scenes, saving you from repetitive code.
2. Server Components: Better Performance, Less JavaScript
React 19 doubles down on Server Components, allowing parts of your UI to render on the server. This reduces the JavaScript sent to users, improving:
- Initial page load speed.
- SEO performance, as server-rendered content is crawler-friendly.
- User experience, with lighter apps and faster interactions.
Use Server Components for pages that fetch data or display static content to boost both speed and SEO rankings.
3. New Hooks for Better Form Management
React 19 introduces new hooks to make form and state management more powerful and developer-friendly:
useFormStatus
: Keeps track of form submission status (e.g., loading or error states).useFormState
: Access the live state of your form fields to build dynamic forms.useOptimistic
: Enables optimistic UI updates, letting you show updates instantly without waiting for server responses.
These hooks are must-haves for apps with forms or dynamic user inputs.
4. React Compiler: Boosting Performance Automatically
The React Compiler is a new addition that optimizes your React code automatically, making your apps faster and reducing the need for manual performance tweaks like memoization.
What it means for you:
- Faster apps out of the box.
- Less time spent debugging performance bottlenecks.
5. Improved Web Components Support
React 19 enhances support for Web Components, making it easier to integrate custom elements into your React projects. This is perfect for teams working with micro frontends or hybrid apps.
6. Simplified Metadata Management
Managing SEO metadata like <title>
and <meta>
tags is now simpler with React 19. Whether it’s dynamic page titles or meta descriptions, this feature makes your app SEO-friendly with less effort.
7. Smarter Asset Loading
React 19 introduces smarter asset loading to improve page transitions. Background loading ensures your app remains smooth and responsive, even on slower networks.
🛠️ Upgrading to React 19
Upgrading to React 19 is straightforward, especially if you're already using React 18.
Steps to Upgrade:
- Update React and React DOM:
npm install react@19 react-dom@19
- Check for deprecated APIs using React 18.3, which highlights issues before upgrading.
- Fix any warnings flagged during the upgrade process. Make sure to test your application thoroughly after upgrading to avoid runtime issues.
🌟 Why React 19 Is a Big Deal
React 19 focuses on making developers' lives easier while enhancing app performance. Here’s why it matters:
- For Developers: New features like Actions and hooks simplify code and reduce boilerplate.
- For Users: Server Components and asset loading make apps faster and more reliable.
- For SEO: Better metadata handling and server rendering improve search rankings.
💡 Final Thoughts
React 19 is a testament to the React team’s commitment to simplifying development while delivering high-performance apps. Whether you’re an experienced developer or just starting out with React, this update offers tools to build faster, smarter, and more scalable applications.
For more React and web development insights, explore my blog.
Learn more on this topic on offical release notes: react.dev