Creating Reusable Components in React: A Comprehensive Guide with Examples
Learn how to create reusable components in React with this comprehensive guide. Explore best practices, step-by-step examples, and tips for efficient and maintainable code. Perfect for developers aiming to streamline their React projects.
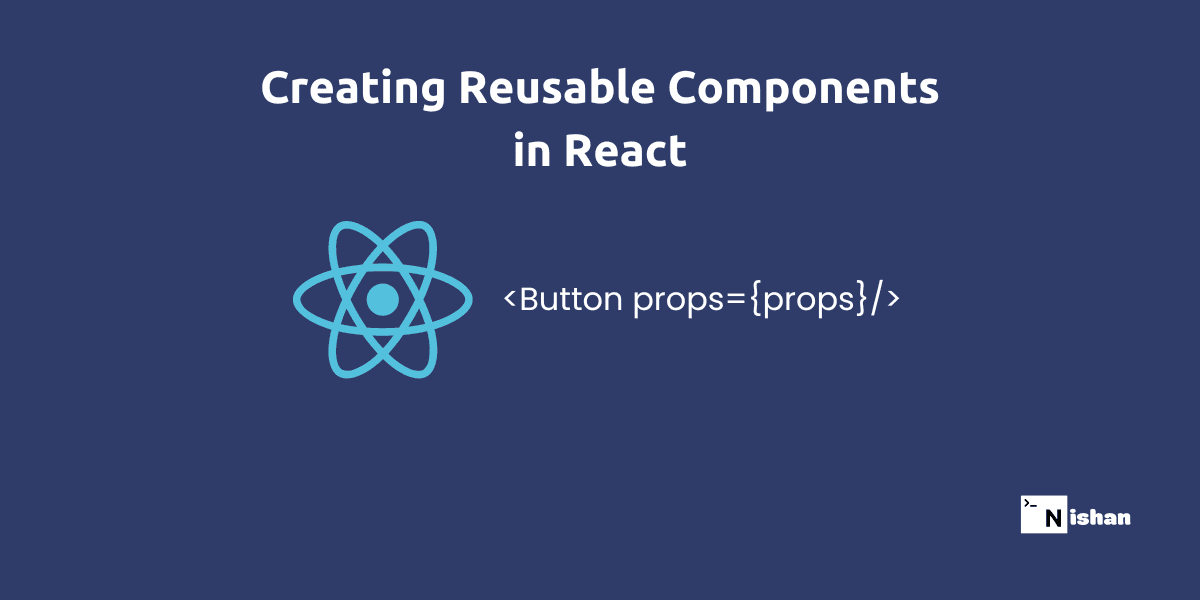
Hi there! 👋 In this guide, we’re going to explore how to build reusable components in React. By the end of this journey, you'll be a pro at creating components that you can use across multiple projects, making your development process faster and more efficient. Let’s dive in!
Table of Contents
What is a React Reusable Component?
A React reusable component is a self-contained module that can be used in different parts of your application without rewriting code. Think of it as a versatile building block that can be configured to serve multiple purposes, enhancing both development speed and code consistency.
Why Reusable Components?
Think of reusable components as the LEGO blocks of your React applications. They offer so many benefits:
Consistency: Your app will have a uniform look and feel.
Efficiency: Save time by not writing the same code over and over.
Scalability: Easily grow your app by using components as building blocks.
Maintainability: Simplify updates and bug fixes with centralized code.
For more on the benefits of reusable components, check out this comprehensive guide on component-based architecture
Principles of Reusable Components
When building reusable components, keep these key principles in mind:
Single Responsibility: Each component should do one thing and do it well.
Composability: Components should be easy to combine with others.
Configurability: Use props to customize behavior without changing the component's code.
Isolation: Avoid side effects that can affect other parts of the app.
Step-by-Step Guide to Building Reusable Components
Component Design
Let’s start simple. Here’s how you can design a basic button component.
import React from "react";
const Button = ({ label, onClick, style, type = "button" }) => {
return (
<button type={type} onClick={onClick} style={style}>
{label}
</button>
);
};
export default Button;
Prop Management
Props are your best friends when it comes to reusable components. They allow you to customize your components effortlessly.
const Button = ({
label,
onClick,
style,
type = "button",
disabled = false,
}) => {
return (
<button type={type} onClick={onClick} style={style} disabled={disabled}>
{label}
</button>
);
};
For a deeper understanding of props, check out the official React documentation on props
Styling
Styling can be handled in many ways. Here’s an example using styled-components.
import styled from "styled-components";
const StyledButton = styled.button`
background-color: #6200ee;
color: white;
padding: 10px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
&:disabled {
background-color: #ccc;
cursor: not-allowed;
}
`;
const Button = ({ label, onClick, disabled = false }) => {
return (
<StyledButton onClick={onClick} disabled={disabled}>
{label}
</StyledButton>
);
};
Learn more about styled-components to enhance your styling techniques.
State Management
For components that need internal state, use hooks. Here’s a simple toggle button example.
import React, { useState } from "react";
const ToggleButton = ({ initialState = false }) => {
const [toggled, setToggled] = useState(initialState);
return (
<button onClick={() => setToggled(!toggled)}>
{toggled ? "On" : "Off"}
</button>
);
};
For more details on managing state with hooks, check out the official React documentation on hooks
Testing and Documentation
Good components are well-tested and documented. Use tools like Jest for testing and Storybook for documentation.
import { render, screen, fireEvent } from "@testing-library/react";
import Button from "./Button";
test("renders button with label", () => {
render(<Button label="Click me" />);
expect(screen.getByText("Click me")).toBeInTheDocument();
});
test("calls onClick handler", () => {
const handleClick = jest.fn();
render(<Button label="Click me" onClick={handleClick} />);
fireEvent.click(screen.getByText("Click me"));
expect(handleClick).toHaveBeenCalledTimes(1);
});
Explore more about Jest and Storybook to enhance your testing and documentation processes.
Best Practices
- Prop Types: Use prop-types or TypeScript for type checking.
- Default Props: Define default values for props to ensure robustness.
- Composition: Design components to be easily composable.
- Documentation: Document usage examples and props clearly.
Conclusion
Building reusable components in React is a game-changer for productivity and code quality. By following the steps and best practices in this guide, you’ll be able to create components that are easy to use and adapt across different projects.
For more insights on React development, check out React’s official blog
Happy coding! 🎉